CORSアクセスの検証のためにaxiosでリクエストを送信する
CORSアクセスの検証のためにaxiosでリクエストを送信する
APIの動作検証のためにaxiosを使ってリクエストを送信する簡易ページを作成します。わざわざaxiosでリクエストを送信する理由はCORSアクセスの動作検証を行うためです。
以下のようなHTMLファイルを作成します。CDNでaxiosをインストールします。
<html>
<head>
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
<script>
async function sendGetRequest() {
try {
const config = {
headers : {
Authorization: "key1"
}
};
const params = {};
const data = await axios.get("http://localhost:8080/items/1", config);
console.log(data);
} catch (e) {
console.log(e);
}
}
async function sendPostRequest() {
try {
const config = {
headers: {
Authorization: "key1"
}
};
const data = {
id: 1,
price: 100,
name: "testItem"
};
const res = await axios.post("http://localhost:8080/items", data, config);
} catch (e) {
console.log(e);
}
}
</script>
</head>
<body>
<button onclick="sendGetRequest()">Send GET Request</button>
<button onclick="sendPostRequest()">Send POST Request</button>
</body>
</html>
このhtmlファイルをhttp-serverでローカルに立てたサーバに配置します。画面はボタンがあるだけです。
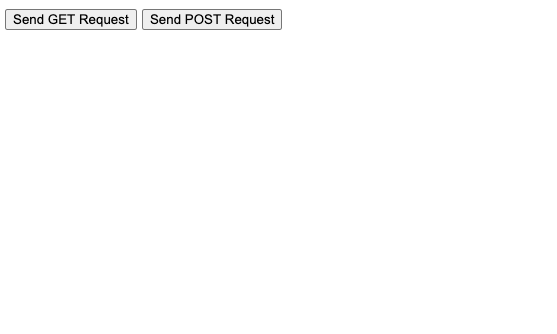
Send GET RequestボタンをクリックするとGETリクエストが送信されます。
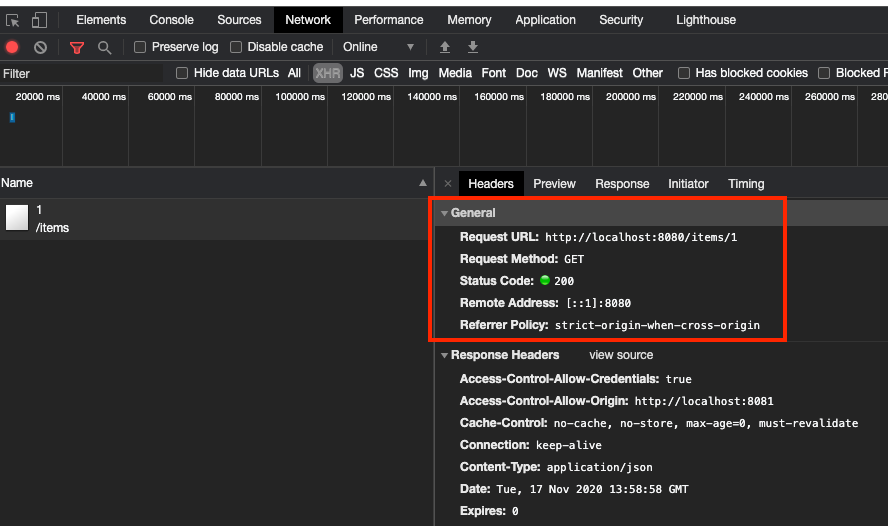